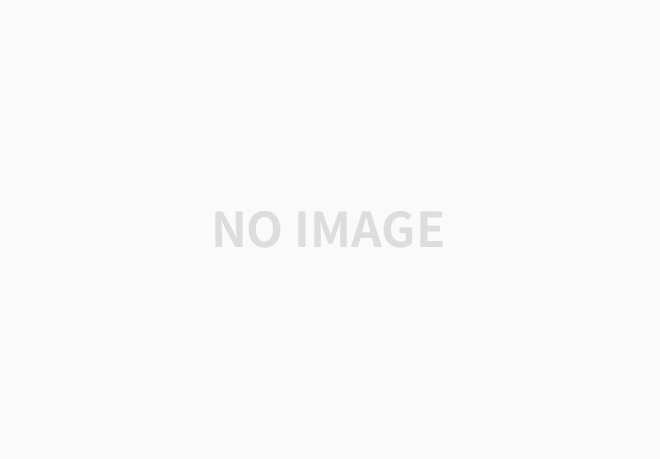
1. DB 연결
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class connection {
public static void main(String[] args) {
-- try 블럭 안에 넣으면 finally 구문에서 연결끊기가 실행 안됨.
Connection conn = null;
try {
-- JDBC Driver를 메모리로 로딩하고, DriverManager에 등록
Class.forName("com.mysql.cj.jdbc.Driver");
-- 연결하기
conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/sample",
"(아이디)",
"(비밀번호)"
);
System.out.println("연결 성공");
} catch (ClassNotFoundException e) {
e.printStackTrace(); -- 예외 종류, 발생 이유, 어디서 발생했는지 추적한 내용까지 출력
} catch (SQLException e) {
e.printStackTrace();
} finally {
if (conn != null) { -- null 이면 굳이 닫을 필요 없기 때문.
try {
/* 연결 끊기 (try 구문 안에 넣으면 예외 발생시 실행이 안되기 때문에
finally 구문에서 무조건 실행되도록 함.) */
conn.close();
System.out.println("연결 끊기");
} catch (SQLException e) {}
}
}
}
}
2. INSERT
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class UserInsert {
public static void main(String[] args) {
Connection conn = null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/sample",
"(아이디)",
"(비밀번호)"
);
System.out.println("연결 성공");
-- 매개변수화된 SQL문 작성
String sql = "" + "INSERT INTO users (
userid, username, userpassword, userage, useremail) " +
"VALUES (?,?,?,?,?)"; // 매개변수에 들어갈 값을 물음표로 표현해준다.
-- PreparedStatement 얻기 및 값 지정
PreparedStatement pstmt = conn.prepareStatement(sql);
-- 연결 객체를 통해 sql문을 실행해서 결과문을 받을 statement를 준비하고,
-- 구현객체가 리턴되어 PreparedStatement 인터페이스 변수에 대입된다.
(아직 실행된 상태는 아니다.)
pstmt.setString(1, "winter");
pstmt.setString(2, "한겨울");
pstmt.setString(3, "12345");
pstmt.setInt(4, 25);
pstmt.setString(5, "winter@mycompany.com");
-- SQL문 실행
int rows = pstmt.executeUpdate(); -- DB에서 insert문을 실행하는 메소드
System.out.println("저장된 행 수: " + rows); -- DB에 반영된 행의 수를 뜻함.
-- 정상적으로 실행되었다면 1이 나옴.
-- PreparedStatement 닫기
pstmt.close();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
if (conn != null) {
try {
-- 연결 끊기
conn.close();
System.out.println("연결 끊기");
} catch (SQLException e) {}
}
}
}
}
3. UPDATE
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Statement;
public class BoardUpdate {
public static void main(String[] args) {
Connection conn = null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/sample",
"(아이디)",
"(비밀번호)"
);
-- 매개변수화된 SQL문 작성
String sql = new StringBuilder() -- 문자열 연결 메서드
.append("UPDATE boards SET ")
.append("btitle=?, ")
.append("bcontent=?, ")
.append("bfilename=?, ")
.append("bfiledata=? ")
.append("WHERE bno=?")
.toString();
-- PreparedStatement 얻기 및 저장
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "눈사람");
pstmt.setString(2, "눈으로 만든 사람");
pstmt.setString(3, "snowman.jpg");
pstmt.setBlob(4, new FileInputStream("src/DBtest/photo2.jpg"));
pstmt.setInt(5, 3); // boards 테이블에 있는 게시물 번호(bno) 지정
-- SQL문 실행
int rows = pstmt.executeUpdate();
System.out.println("수정된 행 수: " + rows);
-- PreparedStatement 닫기
pstmt.close();
} catch (Exception e) {
e.printStackTrace();
} finally {
if(conn != null) {
try {
-- 연결 끊기
conn.close();
} catch (SQLException e) {}
}
}
}
}
4. DELETE
public class BoardDelete {
public static void main(String[] args) {
Connection conn = null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/sample",
"(아이디)",
"(비밀번호)"
);
-- 매개변수화된 SQL문 작성
String sql = "DELETE FROM boards WHERE btitle=?";
-- PreparedStatement 얻기 및 저장
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "눈사람");
-- SQL문 실행
int rows = pstmt.executeUpdate();
System.out.println("삭제된 행 수: " + rows);
-- PreparedStatement 닫기
pstmt.close();
} catch (Exception e) {
e.printStackTrace();
} finally {
if(conn != null) {
try {
-- 연결 끊기
conn.close();
} catch (SQLException e) {}
}
}
}
}
5. SELECT
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import com.mysql.cj.jdbc.Blob;
public class BoardSelect {
public static void main(String[] args) {
Connection conn = null;
try {
-- JDBC Driver 등록
Class.forName("com.mysql.cj.jdbc.Driver");
-- DB 연결
conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/sample",
"(아이디)",
"(비밀번호)");
-- 매개변수화된 SQL문 작성
String sql = "SELECT bno, btitle,bcontent, bwriter,
bdate, bfilename, bfiledata
from boards where bwriter =?";
-- PreparedStatement 얻기 및 값 지정
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1,"winter");
-- SQL문 실행 후, ResultSet을 통해 데이터 읽기.
ResultSet rs = pstmt.executeQuery();
List<Board> boards = new ArrayList<>();
while(rs.next()) {
Board board = new Board(); -- board 테이블의 행을 저장할 객체를 만듦.
board.setBno(rs.getInt("bno"));
board.setBtitle(rs.getString("btitle"));
board.setBcontent(rs.getString("bcontent"));
board.setBwriter(rs.getString("bwriter"));
board.setBdate(rs.getDate("bdate"));
board.setBfilename(rs.getString("bfilename"));
board.setBfileData(rs.getBlob("bfileData"));
System.out.println(board); -- 콘솔에 출력
}
rs.close();
pstmt.close();
} catch (Exception e) {
e.printStackTrace();
} finally {
if (conn != null) {
try {
-- 연결 끊기
conn.close();
} catch (SQLException e) {}
}
}
}
}
[ 내용 참고 : 책 '이것이 자바다' ]
'Database > MySQL' 카테고리의 다른 글
MySQL 새 계정 만들기 (Mac ver) - terminal VS. MySQL 워크벤치 (0) | 2024.07.25 |
---|---|
[MySQL] INNER JOIN / OUTER JOIN / CROSS JOIN / SELF JOIN (2) | 2024.02.25 |
[MySQL] JDBC 정의, 로딩, 프로그램 개발 절차 (0) | 2024.02.25 |
[MySQL] 데이터베이스 모델링 | 참조 무결성 (1) | 2024.02.25 |
[MySQL] 데이터베이스 모델링 | 1~3 정규화, 역정규화 (1) | 2024.02.25 |